Using Assertions in Selenium
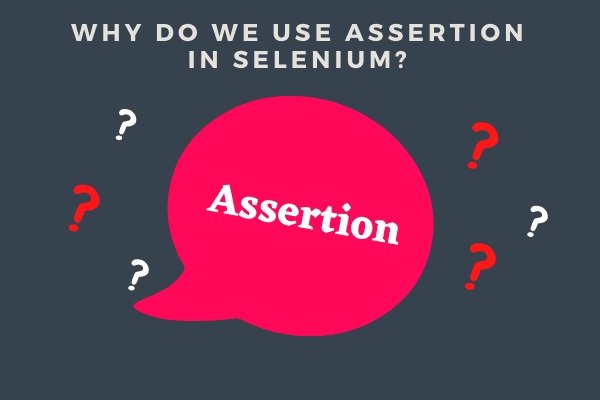
As the software testing field grows, various new concepts are added. You must use a testing framework or tool to perform the software application testing process. There are many such tools available, one of them being Selenium. Selenium is the most popular tool for performing browser automation testing. Selenium is an open source and easy-to-learn tool for testing experts and beginners .
It has multi language support which allows the team to use their preferred language for writing tests . The ultimate goal of selecting an automation testing tool is to make your testing process efficient and productive . There are many such features available in the Selenium testing suite, and one of them is Assertions .
Assertions in Selenium are very useful methods to make your testing process reliable and efficient. It helps the testing team to minimize possible errors. You can compare the two parameters, which are the actual testing result and the expected testing result. In this article, you will see Assertion in Selenium and how to use it.
Introduction to Selenium
Selenium is an open source framework for testing web applications and websites . Selenium can perform automation testing for websites and web applications for various browsers and working frameworks. This framework is a prior choice in testing because it supports many programming languages such as Java, Python, JavaScript, C#, etc. Selenium permits the testing group to perform an iteration of the testing cycle in less time to mechanize the test cases, increasing testing productivity. These features of Selenium make the testing process bug-free and error-free.
Selenium on the cloud is now preferred over using physical infrastructure. Cloud-based Selenium automation testing has many benefits, such as it is economically beneficial, cloud increases the testing scalability, flexibility, and reliability. On the cloud, you can execute faster tests with parallel execution. One such cloud platform is LambdaTest. It is an AI-powered test orchestration and execution platform to run manual and automated tests at scale. The platform allows you to perform real time and automation testing across 3000+ environments and real mobile devices .
Selenium has many components including Selenium IDE Selenium Grid and Selenium WebDriver. IDE helps you to develop your testing code using various programming languages, such as Java Python, etc, just by downloading an extension file for a specific language . With the help of Selenium Grid you can run several test scripts on various machines at once.
It consists of a hub server and numerous grid nodes, with the hub acting as a conduit for commands to reach the registered nodes. Selenium WebDriver allows the tester to communicate directly with the browser for the testing process, which is controlled from the operating system (OS) level. You can create and execute the test cases using it.
What are Assertions?
All the automation testing frameworks have the option to provide a mechanism where the developers and the testing team can raise an assert. The general concept of applying assertions in automation testing is similar in all the testing frameworks and tools. Here, we are specifically discussing Selenium assertions. Assertions are defined as a state of software application that suggests whether your testing meets the expectations and requirements. If the assertions fail for any reason, the test case fails, and the testing execution stops immediately. Apart from that, Selenium assertions can also be used to perform many types of test case validation.
Types of Assertions in Selenium
In Selenium, there are two assertions for automation testing. These two assertions are defined based on the behavior of the assertions and how they respond when a testing condition gets passed or fails. Let us see the two types of assertions in Selenium below:
Hard Assertion
Hard Assertion in Selenium is defined as the assertion that throws the “AssertException” when the automation test fails due to any reason. While performing the Selenium automation testing, if you get a hard assertion issue, you can use the error using a catch block. You need to use this similarly as you use this in exception. For example, suppose you have two test cases in your automation test suite. If the first test fails and has an assertion init, you want to run the second case in the automation test suite. Then, you first need to handle this assertion error. You can see various assertion methods in the given section.
The various methods in Hard Assertion are as follows:
- AssertEquals
- AssertNotEquals
- AssertTrue
- AssertFalse
- AssertNull
- AssertNotNull
AssertEquals: It is an assertion method that is used to compare two parameters. One is the actual result of the testing, and the other is the expected result of automation testing. It returns a boolean output. This method required at least two arguments to work. If both the parameters i.e. expected result and the actual result are the same then the method returns “True” otherwise returns “False”.
import org.junit.Assert;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class AssertionEquals {
public static void main(String[] args) {
System.setProperty(“webdriver.chrome.driver”, “../Dependencies/chromedriver”);
WebDriver webdriver = new ChromeDriver();
webdriver.navigate().to(“https://example.com/”);
String ActualTitle = webdriver.getTitle();
String ExpectedTitle = “This is a example text”;
Assert.assertEquals(ExpectedTitle, ActualTitle);
AssertNotEquals: As the name suggests, this method is the opposite of the assertEqual method. It also requires two parameters: one is the actual result, and the other is the expected result. If the assertion condition is satisfied, but the two are not the same, then this assertion method gets passed with no exception in your automation testing. If the actual and expected results are the same, then this method fails with an exception, and the automation test fails. Given Below is an example to understand this method:
package mypack;
import org.junit.Assert;
public class Checkbox_test {
public static void main(String[] args) {
// TODO Auto-generated method stub
Assert.assertNotEquals(“Hello”, “How are you”);
System.out.println(“Hello…This is example”);
AsserTrue(): This assert method provides a boolean result. Suppose for example the testing result gets passed. Then this assert method will return the boolean value “True”. Below is an example to better understand this:
import org.junit.Assert;
import static org.testng.Assert.assertTrue;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class AssertionEquals {
@Test
public void testAssertFunction(){
System.setProperty(“webdriver.chrome.driver”, “../Dependencies/chromedriver”);
WebDriver webdriver = new ChromeDriver();
webdriver.navigate().to(“https://www.example.com/”);
Boolean verifyTitle = webdriver.getTitle().equalsIgnoreCase(“This is a sample example”);
assertTrue(verifyTitle);
AssertFalse(): As the name suggests, the assertFalse() is the opposite of the assertTrue() method. This method checks if the condition’s Boolean value is true. The assertion method succeeds in the test case if the testing output value is “false”.
AssertNull(): This Selenium assert method is used to verify if the object provided in the automation test is Null. If the object is Null, then the assertion method passes the automation test, and the test gets marked as “pass”. If the provided object in the test is not null, then the test gets marked as “failed”. Below is an example:
package mypack;
import org.junit.Assert;
public class Checkbox_test {
public static void main(String[] args) {
Assert.assertNull(null);
System.out.println(“Hello…this is an example”);
AssertNotNull(): This Selenium assert method is also used to verify if the object provided in the automation test is Null or NotNull. But it works opposite to the assertNull() method. If the provided object of the test is not null, then the test gets passed. If the object is null, then the test fails. In such cases, the method immediately stops the testing process and marks the test as “failed”. Below is an example of this method:
package mypack;
import org.junit.Assert;
public class Checkbox_test
public static void main(String[] args) {
// TODO Auto-generated method stub
Assert.assertNotNull(10);
System.out.println(“C Language”);
Soft Assertion
Now, you are aware of the hard assertion in Selenium. If the error is not tackled with a hard assertion, we can use the soft assertion in Selenium. Soft assertions do not throw any exception on assertion failure unless you ask for it. This is very helpful if you use multiple validations in a form. In the form, only a few validations directly affect the test cases. If both are true, the assertion is true, and the test case is complete.
Best Practices for Using Assertions in Selenium
When performing automation testing, following the best practices to get the maximum yield from your testing is necessary. So, let us see some of the best practices for using assertions in Selenium.
Use Descriptive Test Name
The methods associated with your automation test must be descriptive. It should reflect the workings of the test, what it will do, and its functions. It will make it easy for people to understand the test’s purpose and debug issues when they arise.
Use Assertions for Result Validation
The Selenium assertions can also be used to validate the testing output. Assertions ensure that the testing is according to the expectation or that the tests are giving the result as intended and the software application is working correctly.
Use Data Driven Testing
Data-driven testing is the process in which you use various data sets to test the same functionality of a software application. This ensures that our application functions correctly for different data sets we have taken as input values. It also helps to identify any issues that arise for specific input values.
Use Soft Assertion When Needed Only
Soft assertions are recommended to use in situations when you want to execute all the assertions in one go. Even if one test fails, you can execute all the assertions in the test case. It helps the testing team identify any issues during testing, and you can fix them before releasing them into production.
Conclusion
Assertions in Selenium make your testing process more reliable by comparing two parameters. One is the expected outcome, and the other is the actual outcome of the testing process. Selenium assertions are basically of two types: hard assertion and soft assertion. Hope you got an idea of how to use the Selenium assertion after reading this article.